This project was a group project for the University of California Riverside software construction course. A software engineer needs skills in agile, software design, design patterns, and version control. For me, the rshell project was another step in acquiring these skills.
Skills Learned
Agile Methodology
Although we learned about Scrum and Kanban during class, we used the lean Kanban approach. We visualized our work, limited our work in progress, tracked our work flow, made management policies, and improved collaboratively. Since we were a two person team, this approach worked better for us.
We wrote a design document as a starting point for the design of the rshell software. We then met during weekly sprints to discuss, design, and code the feature for that week. Finally, we submitted the finished feature and received feedback and repeated this process for the next feature.
Design Pattern and Program Design
We used the strategy pattern to implement the rshell program. Why the strategy pattern? It allowed us to implement the related algorithms of the different connectors. One great advantage is that we were able to decouple the commands from the call structure of the shell.
The abstract base class, “Base”, was the compositor and enforced the interface on the derived Connector and Command classes. The connector classes served as the concrete strategy to separate data from algorithm. This is a snapshot from the documentation generated using doxygen.
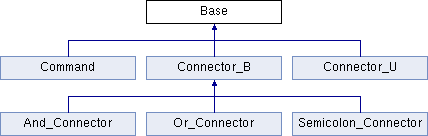
A parser class was used to parse the user input and generate a vector of tokens to build a tree of commands using the tree class. The tree was built from command objects as leaves and, if there were connectors, subtrees created from command tree objects. Each node consisted of base class pointers. The command tree class served as the context in our program. Here is a doxygen inheritance graph of the command tree class

Version Control
We used Git for version control and hosted our repository on Github. The gitignore file we wrote filtered out compiled source, log and database files, operating specific files, and IDE generated files. We created new branches as we worked on features and merged with the master branch once the feature was completed and the bugs were worked out. Lastly, we used tags to specify the version of the software. Since this project encompassed various assignments, the tags are for homework 2 through 4, which are versions 1 through 3 of the software.
Debugging and Testing
GDB served as our main debugger in combination with the occasional output message to std error and std out for debugging. We also used valgrind to double check for memory leaks, particularly when working on the destructor for the command tree and tree classes. The functions and features were tested out during weekly sprints. We wrote bash scripts to test the functionality of our program.
Observations and Future Plans
This was my first project where the focus was on the agile methodology, git, and design patterns. It was a valuable experience because I learned to program in tight collaboration as part of a group. It was also great to collaborate with a brilliant young software engineer.
The bug with the test command listed on my github repository had not been addressed yet. However, I plan on integrating this with a project from my concurrent systems course as time allows. This would involve allowing commands to run in the background, foreground, and several other features from my concurrent systems course.